Adding / Modifying field validators
#
Step 1: Front EndNow that you have added new fields to your signup form, let's see how you can add validators to make sure that your users provide the right information.
You can add a validate
method to any formFields
.
Building up on our previous example, let's add an age verification to our form:
- ReactJS
- Angular
- Vue
- Plain JavaScript
- React Native
Note
You can use the
You can refer to this example app as a reference for using the
supertokens-web-js
SDK which exposes several helper functions that query the APIs exposed by the SuperTokens backend SDK.You can refer to this example app as a reference for using the
supertokens-web-js
SDK.Note
To use SuperTokens with React Native you need to use the
To add login functionality, you need to build your own UI and call the APIs exposed by the backend SDKs. You can find the API spec here
supertokens-react-native
SDK. The SDK provides session management features.To add login functionality, you need to build your own UI and call the APIs exposed by the backend SDKs. You can find the API spec here
What type of UI are you using?
Prebuilt UICustom UI
What type of UI are you using?
Prebuilt UICustom UI
import SuperTokens from "supertokens-auth-react";
import ThirdPartyEmailPassword from "supertokens-auth-react/recipe/thirdpartyemailpassword";
import Session from "supertokens-auth-react/recipe/session";
SuperTokens.init({
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "..."
},
recipeList: [
ThirdPartyEmailPassword.init({
signInAndUpFeature: {
signUpForm: {
formFields: [{
id: "name",
label: "Full name",
placeholder: "First name and last name"
}, {
id: "age",
label: "Your age",
placeholder: "How old are you?",
optional: true,
/* Validation method to make sure that age is above 18 */
validate: async (value) => {
if (parseInt(value) > 18) {
return undefined; // means that there is no error
}
return "You must be over 18 to register";
}
}, {
id: "country",
label: "Your country",
placeholder: "Where do you live?",
optional: true
}]
}
}
}),
Session.init()
]
});
Here is what happens if someone tries to register with an age of 17:
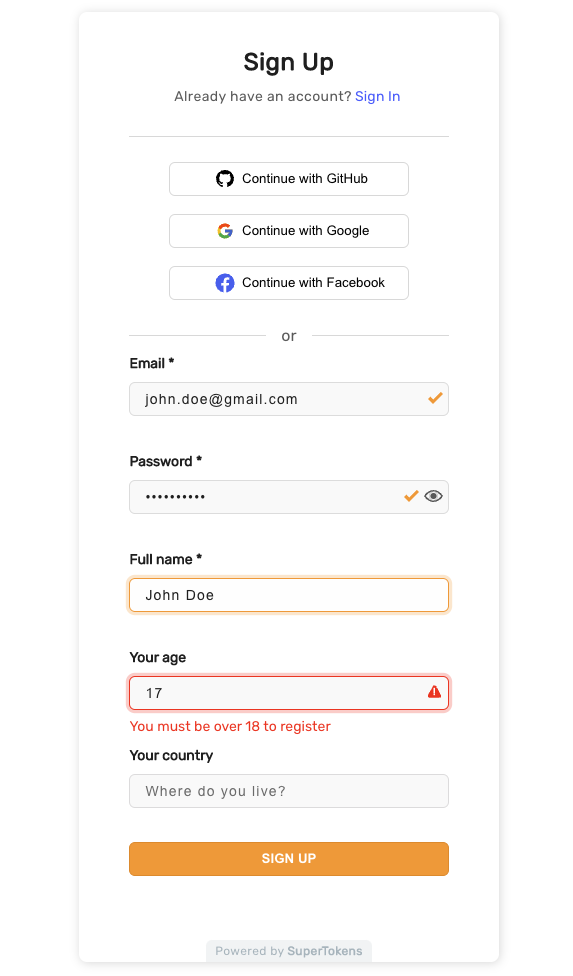
Security
Front-end validation is great for user experience but you should always make sure that you are also applying these validations on the backend.
#
Step 2: Back EndIn your backend's SuperTokens init method, let's replicate the validate
functions from above:
- NodeJS
- GoLang
- Python
import SuperTokens from "supertokens-node";
import ThirdPartyEmailPassword from "supertokens-node/recipe/thirdpartyemailpassword";
import Session from "supertokens-node/recipe/session";
SuperTokens.init({
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "..."
},
supertokens: {
connectionURI: "...",
},
recipeList: [
ThirdPartyEmailPassword.init({
signUpFeature: {
formFields: [{
id: "name"
}, {
id: "age",
/* Validation method to make sure that age >= 18 */
validate: async (value) => {
if (parseInt(value) >= 18) {
return undefined; // means that there is no error
}
return "You must be over 18 to register";
}
}, {
id: "country",
optional: true
}]
}
}),
Session.init({
})
]
});
import (
"strconv"
"github.com/supertokens/supertokens-golang/recipe/emailpassword/epmodels"
"github.com/supertokens/supertokens-golang/recipe/thirdpartyemailpassword"
"github.com/supertokens/supertokens-golang/recipe/thirdpartyemailpassword/tpepmodels"
"github.com/supertokens/supertokens-golang/supertokens"
)
func main() {
countryOptional := true
supertokens.Init(supertokens.TypeInput{
RecipeList: []supertokens.Recipe{
thirdpartyemailpassword.Init(&tpepmodels.TypeInput{
SignUpFeature: &epmodels.TypeInputSignUp{
FormFields: []epmodels.TypeInputFormField{
{
ID: "name",
},
{
ID: "age",
Validate: func(value interface{}) *string {
age, _ := strconv.Atoi(value.(string))
if age >= 18 {
// return nil to indicate success
return nil
}
err := "You must be over 18 to register"
return &err
},
},
{
ID: "country",
Optional: &countryOptional,
},
},
},
}),
},
})
}
from supertokens_python import init, InputAppInfo
from supertokens_python.recipe import thirdpartyemailpassword
from supertokens_python.recipe.emailpassword import InputFormField
from typing import Any
async def validate_age(value: Any):
# Validation method to make sure that age >= 18
if int(value) >= 18:
return None # means that there is no error
return 'You must be over 18 to register'
init(
app_info=InputAppInfo(api_domain="...", app_name="...", website_domain="..."),
framework='...',
recipe_list=[
thirdpartyemailpassword.init(
sign_up_feature=thirdpartyemailpassword.InputSignUpFeature(
form_fields=[
InputFormField(id='name'),
InputFormField(id='age', validate=validate_age),
InputFormField(id='country', optional=True)
]
)
)
]
)
#
Changing the default email and password validatorsBy default, SuperTokens adds an email and a password validator to the sign-up form:
- The default email validator makes sure that the provided email is in the correct email format.
- The default password validator makes sure that the provided password:
- has a minimum of 8 characters.
- contains at least one lowercase character
- contains at least one number
important
- The email validator that you define for Sign up is also applied automatically to Sign In.
- The password validator that you define for Sign up is also applied automatically to reset password forms.
#
Step 1: Front End 🚪- ReactJS
- Angular
- Vue
- Plain JavaScript
- React Native
Note
You can use the
You can refer to this example app as a reference for using the
supertokens-web-js
SDK which exposes several helper functions that query the APIs exposed by the SuperTokens backend SDK.You can refer to this example app as a reference for using the
supertokens-web-js
SDK.Note
To use SuperTokens with React Native you need to use the
To add login functionality, you need to build your own UI and call the APIs exposed by the backend SDKs. You can find the API spec here
supertokens-react-native
SDK. The SDK provides session management features.To add login functionality, you need to build your own UI and call the APIs exposed by the backend SDKs. You can find the API spec here
What type of UI are you using?
Prebuilt UICustom UI
What type of UI are you using?
Prebuilt UICustom UI
import SuperTokens from "supertokens-auth-react";
import ThirdPartyEmailPassword from "supertokens-auth-react/recipe/thirdpartyemailpassword";
SuperTokens.init({
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "..."
},
recipeList: [
ThirdPartyEmailPassword.init({
signInAndUpFeature: {
signUpForm: {
formFields: [{
id: "email",
label: "...",
validate: async (value) => {
// Your own validation returning a string or undefined if no errors.
return "...";
}
}, {
id: "password",
label: "...",
validate: async (value) => {
// Your own validation returning a string or undefined if no errors.
return "...";
}
}]
}
}
})
]
});
#
Step 2: Back End 📫- NodeJS
- GoLang
- Python
import SuperTokens from "supertokens-node";
import ThirdPartyEmailPassword from "supertokens-node/recipe/thirdpartyemailpassword";
SuperTokens.init({
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "..."
},
supertokens: {
connectionURI: "...",
},
recipeList: [
ThirdPartyEmailPassword.init({
signUpFeature: {
formFields: [{
id: "email",
validate: async (value) => {
// Your own validation returning a string or undefined if no errors.
return "...";
}
}, {
id: "password",
validate: async (value) => {
// Your own validation returning a string or undefined if no errors.
return "...";
}
}]
}
}),
]
});
import (
"github.com/supertokens/supertokens-golang/recipe/emailpassword/epmodels"
"github.com/supertokens/supertokens-golang/recipe/thirdpartyemailpassword"
"github.com/supertokens/supertokens-golang/recipe/thirdpartyemailpassword/tpepmodels"
"github.com/supertokens/supertokens-golang/supertokens"
)
func main() {
supertokens.Init(supertokens.TypeInput{
RecipeList: []supertokens.Recipe{
thirdpartyemailpassword.Init(&tpepmodels.TypeInput{
SignUpFeature: &epmodels.TypeInputSignUp{
FormFields: []epmodels.TypeInputFormField{
{
ID: "email",
Validate: func(value interface{}) *string {
// Your own validation returning a string or nil if no errors.
return nil
},
},
{
ID: "password",
Validate: func(value interface{}) *string {
// Your own validation returning a string or nil if no errors.
return nil
},
},
},
},
}),
},
})
}
from supertokens_python import init, InputAppInfo
from supertokens_python.recipe import thirdpartyemailpassword
from supertokens_python.recipe.emailpassword import InputFormField
from typing import Any
async def validate_password(value: Any):
pass # TODO
async def validate_email(value: Any):
pass # TODO
init(
app_info=InputAppInfo(api_domain="...", app_name="...", website_domain="..."),
framework='...',
recipe_list=[
thirdpartyemailpassword.init(
sign_up_feature=thirdpartyemailpassword.InputSignUpFeature(
form_fields=[
InputFormField(id='password', validate=validate_password),
InputFormField(id='email', validate=validate_email)
]
)
)
]
)