Enable email verification
important
Email verification is turned off by default. It is strongly encouraged to enable it to ensure the authenticity of your users.
There are two modes of email verification:
REQUIRED
OPTIONAL
When you enable this, you will need to provide the mode.
REQUIRED
vs OPTIONAL
modes#
The REQUIRED
#
Mode set to - On the backend:
- It adds info about if the email is verified or not in the session claims during session creation.
- Calls to
verifySession()
orgetSession
will return an error if email verification is not completed.
- On the frontend:
- If using React and using ` wrapper: It will redirect the user to the email verification page if the email verification check fails.
- If not using React, or not using
<SessionAuth />
: You can check if the email verification claim istrue
or not and take action based on that.
OPTIONAL
#
Mode set to - On the backend: It adds info about if the email is verified or not in the session claims during session creation.
- On the frontend:
- If using React and using
<SessionAuth />
wrapper: It adds information about if email verification claim istrue
or not in the session context object. It's up to you to decide what to do in your routes based on this information. - If not using React, or not using
<SessionAuth />
: You can check if the email verification claim istrue
or not and take action based on that.
- If using React and using
#
Backend setup- NodeJS
- GoLang
- Python
import SuperTokens from "supertokens-node";
import EmailVerification from "supertokens-node/recipe/emailverification";
import Session from "supertokens-node/recipe/session";
SuperTokens.init({
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "...",
},
recipeList: [
EmailVerification.init({
mode: "REQUIRED",
}),
Session.init(),
],
});
import (
"github.com/supertokens/supertokens-golang/recipe/emailverification"
"github.com/supertokens/supertokens-golang/recipe/emailverification/evmodels"
"github.com/supertokens/supertokens-golang/recipe/session"
"github.com/supertokens/supertokens-golang/recipe/session/sessmodels"
"github.com/supertokens/supertokens-golang/supertokens"
)
func main() {
supertokens.Init(supertokens.TypeInput{
RecipeList: []supertokens.Recipe{
emailverification.Init(evmodels.TypeInput{
Mode: evmodels.ModeRequired,
}),
session.Init(&sessmodels.TypeInput{}),
},
})
}
from supertokens_python import init, InputAppInfo
from supertokens_python.recipe import session
from supertokens_python.recipe import emailverification
init(
app_info=InputAppInfo(
api_domain="...", app_name="...", website_domain="..."),
framework='...',
recipe_list=[
emailverification.init(mode='REQUIRED'),
session.init()
]
)
#
Frontend setup- ReactJS
- Angular
- Vue
- Plain JavaScript
- React Native
Note
To use SuperTokens with React Native you need to use the
To add login functionality, you need to build your own UI and call the APIs exposed by the backend SDKs. You can find the API spec here
supertokens-react-native
SDK. The SDK provides session management features.To add login functionality, you need to build your own UI and call the APIs exposed by the backend SDKs. You can find the API spec here
import SuperTokens from "supertokens-auth-react";
import EmailVerification from "supertokens-auth-react/recipe/emailverification";
import Session from "supertokens-auth-react/recipe/session";
SuperTokens.init({
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "...",
},
recipeList: [
EmailVerification.init({
mode: "REQUIRED",
}),
Session.init(),
],
});
What type of UI are you using?
Prebuilt UICustom UI
What type of UI are you using?
Prebuilt UICustom UI
- Via NPM
- Via Script Tag
import SuperTokens from 'supertokens-web-js';
import Session from 'supertokens-web-js/recipe/session';
import EmailVerification from "supertokens-web-js/recipe/emailverification";
SuperTokens.init({
appInfo: {
apiDomain: "...",
apiBasePath: "...",
appName: "...",
},
recipeList: [
EmailVerification.init(),
Session.init(),
],
});
supertokens.init({
appInfo: {
apiDomain: "...",
apiBasePath: "...",
appName: "...",
},
recipeList: [
supertokensEmailVerification.init(),
supertokensSession.init(),
],
});
#
The default UI (Pre built UI only)When a new user signs up with an unverified email, they will receive an email to verify their address and be redirected to the following screen:
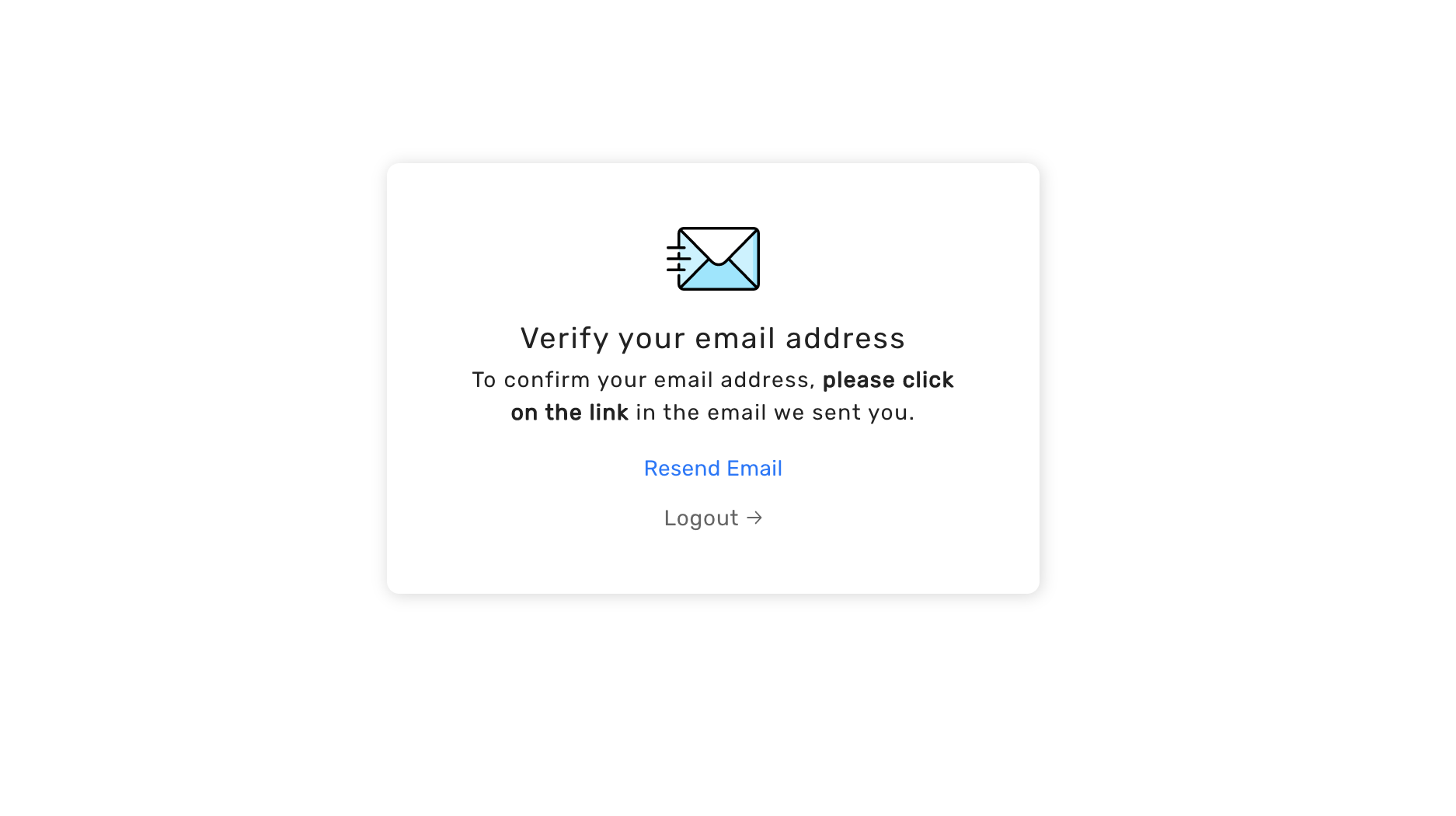
After they have clicked on the email, they will see this screen:
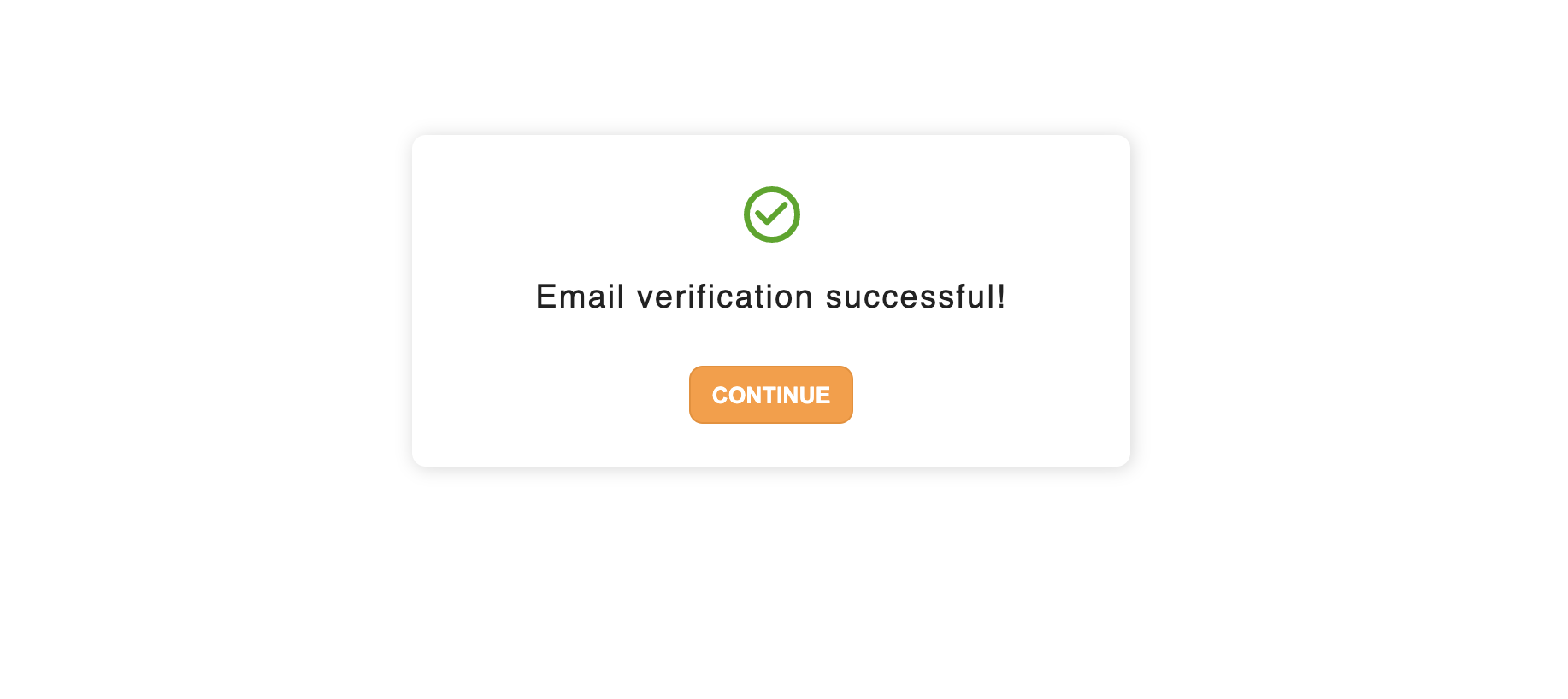