Frontend Integration
#
Supported frameworksfor mobile apps
For mobile apps, please see the custom UI section (see left nav bar)
Automatic setup using CLI
Run the following command in your terminal.
npx create-supertokens-app@latest --recipe=emailpassword
Once this is done, you can skip Step (1) and (2) in this section (see left nav bar) and move directly to setting up the SuperTokens core (Step 3).
Or, you can manually integrate SuperTokens by following the steps below.
Manual setup steps below
#
1) Install- ReactJS
- Angular
- Vue
npm i -s supertokens-auth-react
important
SuperTokens does not provide Angular components for pre built UI, but, there are two ways of implementing SuperTokens in your Angular frontend:
- Load SuperTokens's pre-built React UI components in your Angular app. Continue following the guide for this flow.
- Build your own UI. Click here to learn more.
Start by installing the SuperTokens ReactJS SDK and React:
npm i -s supertokens-auth-react react react-dom
You will also need to update compilerOptions
in your tsconfig.json
file with the following:
"jsx": "react",
"allowSyntheticDefaultImports": true,
important
SuperTokens does not provide Vue components for pre built UI, but, there are two ways of implementing SuperTokens in your Vue frontend:
- Load SuperTokens's pre-built React UI components in your Vue app. Continue following the guide for this flow.
- Build your own UI. Click here to learn more.
Start by installing the SuperTokens ReactJS SDK and React:
npm i -s supertokens-auth-react react react-dom
init
function#
2) Call the - ReactJS
- Angular
- Vue
In your App.js
file, import SuperTokens and call the init
function
import React from 'react';
import SuperTokens, { SuperTokensWrapper } from "supertokens-auth-react";
import EmailPassword from "supertokens-auth-react/recipe/emailpassword";
import Session from "supertokens-auth-react/recipe/session";
SuperTokens.init({
appInfo: {
// learn more about this on https://supertokens.com/docs/emailpassword/appinfo
appName: "<YOUR_APP_NAME>",
apiDomain: "<YOUR_API_DOMAIN>",
websiteDomain: "<YOUR_WEBSITE_DOMAIN>",
apiBasePath: "/auth",
websiteBasePath: "/auth",
},
recipeList: [
EmailPassword.init(),
Session.init()
]
});
/* Your App */
class App extends React.Component {
render() {
return (
<SuperTokensWrapper>
{/*Your app components*/}
</SuperTokensWrapper>
);
}
}
Before we initialize the supertokens-auth-react
SDK let's see how we will use it in our Angular app
Architecture
- The
supertokens-auth-react
SDK is responsible for rendering the login UI, handling authentication flows and session management. - We could use this SDK for the entire app but it would inflate the bundle size with UI components and dependencies that most of our app won't use.
- That's where the
supertokens-web-js
SDK comes in. It contains the functions to handle authentication and session management without any of the pre-built UI. In fact, thesupertokens-web-js
SDK is used internally by thesupertokens-auth-react
SDK. - We can now reduce the bundle size and load times for all non-auth-related routes in our app by only initialising the
supertokens-auth-react
SDK for auth-related routes and use thesupertokens-web-js
SDK for session management in the rest of our app.
Creating the /auth
route
Use the Angular CLI to generate a new route
ng generate module auth --route auth --module app.module
In your
auth
component folder create a react componentsupertokensAuthComponent.tsx
where we initialize thesupertokens-auth-react
SDK. This will tell SuperTokens which UI to show when the user visits the login page./app/auth/supertokensAuthComponent.tsximport * as React from "react";
import * as SuperTokens from "supertokens-auth-react";
import * as EmailPassword from "supertokens-auth-react/recipe/emailpassword";
import Session from "supertokens-auth-react/recipe/session";
SuperTokens.init({
appInfo: {
appName: "<YOUR_APP_NAME>",
apiDomain: "<YOUR_API_DOMAIN>",
websiteDomain: "<YOUR_WEBSITE_DOMAIN>",
apiBasePath: "/auth",
websiteBasePath: "/auth"
},
recipeList: [
EmailPassword.init(),
Session.init(),
],
});
class SuperTokensReactComponent extends React.Component {
override render() {
if (SuperTokens.canHandleRoute()) {
return SuperTokens.getRoutingComponent();
}
return "Route not found";
}
}
export default SuperTokensReactComponent;Load
superTokensAuthComponent
in theauth
angular component/app/auth/auth.component.tsimport { AfterViewInit, Component, OnDestroy } from "@angular/core";
import * as React from "react";
import * as ReactDOM from "react-dom";
import SuperTokensReactComponent from "./supertokensAuthComponent.tsx";
@Component({
selector: "app-auth",
template: '<div [id]="rootId"></div>',
})
export class AuthComponent implements AfterViewInit, OnDestroy {
title = "angularreactapp";
public rootId = "rootId";
// We use the ngAfterViewInit lifecycle hook to render the React component after the Angular component view gets initialized
ngAfterViewInit() {
ReactDOM.render(React.createElement(SuperTokensReactComponent), document.getElementById(this.rootId));
}
// We use the ngOnDestroy lifecycle hook to unmount the React component when the Angular wrapper component is destroyed.
ngOnDestroy() {
ReactDOM.unmountComponentAtNode(document.getElementById(this.rootId) as Element);
}
}Initialize the
supertokens-web-js
SDK in your angular app's root component. This will provide session management across your entire application./app/app.component.tsimport { Component } from "@angular/core";
import * as SuperTokens from "supertokens-web-js";
import * as Session from "supertokens-web-js/recipe/session";
SuperTokens.init({
appInfo: {
appName: "<YOUR_APP_NAME>",
apiDomain: "<YOUR_API_DOMAIN>",
},
recipeList: [Session.init()],
});
@Component({
selector: "app-root",
template: "<router-outlet></router-outlet>",
})
export class AppComponent {
title = "<YOUR_APP_NAME>";
}
Before we initialize the supertokens-auth-react
SDK let's see how we will use it in our Vue app
Architecture
- The
supertokens-auth-react
SDK is responsible for rendering the login UI, handling authentication flows and session management. - We could use this SDK for the entire app but it would inflate the bundle size with UI components and dependencies that most of our app won't use.
- That's where the
supertokens-web-js
SDK comes in. It contains the functions to handle authentication and session management without any of the pre-built UI. In fact, thesupertokens-web-js
SDK is used internally by thesupertokens-auth-react
SDK. - We can now reduce the bundle size and load times for all non-auth-related routes in our app by only initialising the
supertokens-auth-react
SDK for auth-related routes and use thesupertokens-web-js
SDK for session management in the rest of our app.
Creating the /auth
route
Create a new file
AuthView.vue
, this Vue component will be used to render the auth componentCreate a React component
Supertokens.tsx
where we initialize thesupertokens-auth-react
SDK. This will tell SuperTokens which UI to show when the user visits the login page./components/Supertokens.tsximport * as SuperTokens from "supertokens-auth-react";
import * as EmailPassword from "supertokens-auth-react/recipe/emailpassword";
import Session from "supertokens-auth-react/recipe/session";
SuperTokens.init({
appInfo: {
appName: "<YOUR_APP_NAME>",
apiDomain: "<YOUR_API_DOMAIN>",
websiteDomain: "<YOUR_WEBSITE_DOMAIN>",
apiBasePath: "/auth",
websiteBasePath: "/auth"
},
recipeList: [
EmailPassword.init(),
Session.init(),
],
});
function SuperTokensReactComponent(props: any) {
if (SuperTokens.canHandleRoute()) {
return SuperTokens.getRoutingComponent();
}
return "Route not found";
}
export default SuperTokensReactComponent;Load
SuperTokensReactComponent
in theAuthView.vue
component/views/AuthView.vue<script lang="ts">
import * as React from "react";
import * as ReactDOM from "react-dom";
import SuperTokensReactComponent from "../components/Supertokens";
export default {
mounted: () => {
ReactDOM.render(
React.createElement(SuperTokensReactComponent as React.FC),
document.getElementById("authId")
);
}
};
</script>
<template>
<main>
<div id="authId" />
</main>
</template>Initialize the
supertokens-web-js
SDK in your Vue app'smain.ts
file. This will provide session management across your entire application./main.tsimport { createApp } from "vue";
import * as SuperTokens from "supertokens-web-js";
import * as Session from "supertokens-web-js/recipe/session";
import App from "./App.vue";
import router from "./router";
SuperTokens.init({
appInfo: {
appName: "<YOUR_APP_NAME>",
apiDomain: "<YOUR_API_DOMAIN>",
},
recipeList: [Session.init()],
});
const app = createApp(App);
app.use(router);
app.mount("#app");
#
3) Setup Routing to show the login UI- ReactJS
- Angular
- Vue
Update your angular router so that all auth related requests load the auth
component
import { NgModule } from "@angular/core";
import { RouterModule, Routes } from "@angular/router";
const routes: Routes = [
{
path: "auth",
loadChildren: () => import("./auth/auth.module").then((m) => m.AuthModule),
},
{ path: "**", loadChildren: () => import("./home/home.module").then((m) => m.HomeModule) },
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule],
})
export class AppRoutingModule {}
Update your Vue router so that all auth related requests load the AuthView
component
import { createRouter, createWebHistory } from "vue-router";
import HomeView from "../views/HomeView.vue";
const router = createRouter({
history: createWebHistory(import.meta.env.BASE_URL),
routes: [
{
path: "/",
name: "home",
component: HomeView,
},
{
path: "/auth*",
name: "auth",
component: () => import("../views/AuthView.vue"),
},
],
});
export default router;
#
4) View the login UIYou can view the login UI by visiting /auth
.
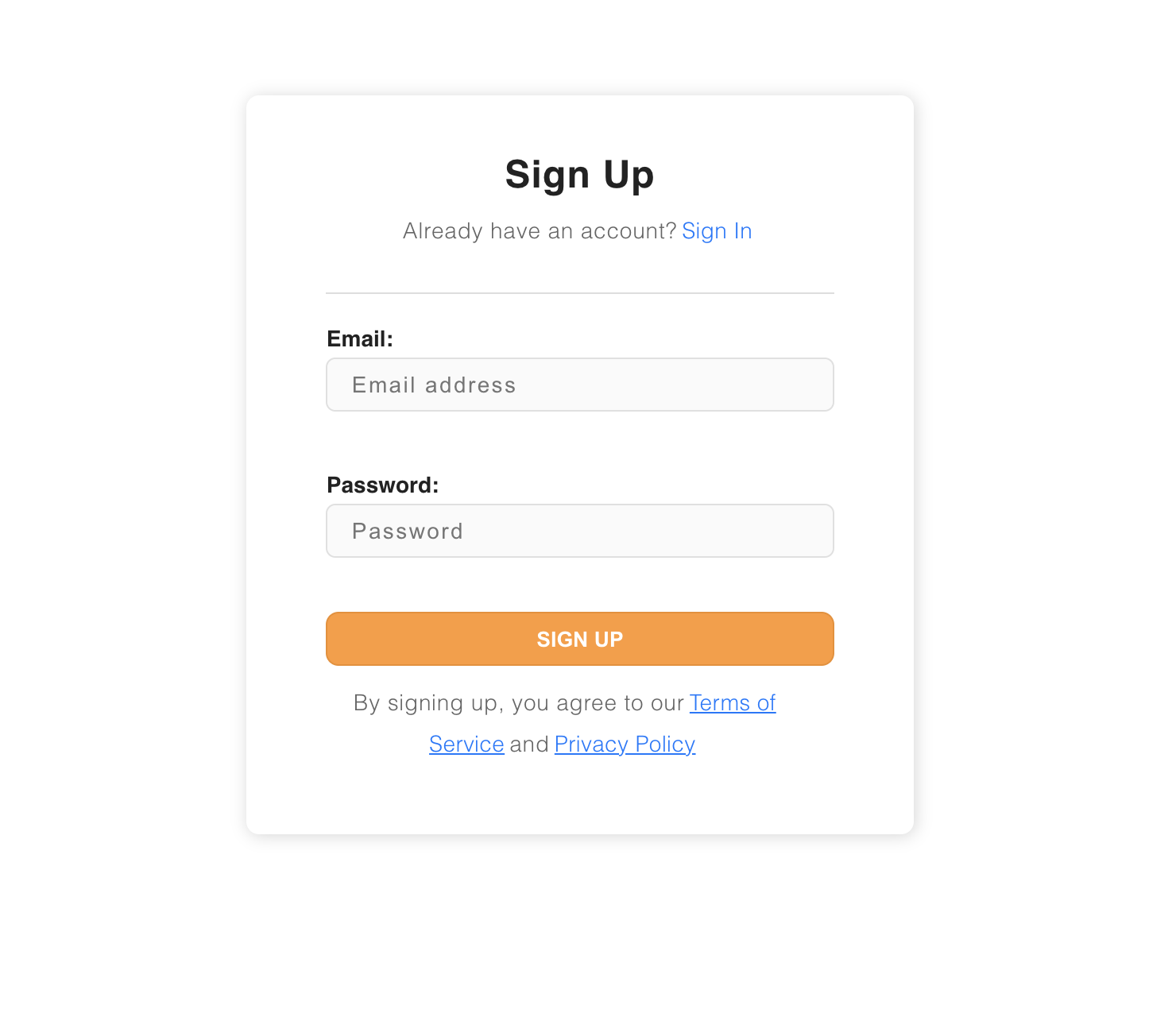
At this stage, you've successfully integrated your website with SuperTokens. The next section will guide you through setting up your backend.