Frontend Integration
#
Supported frameworksfor mobile apps
For mobile apps, please see the custom UI section (see left nav bar)
Automatic setup using CLI
Run the following command in your terminal.
npx create-supertokens-app@latest --recipe=thirdpartypasswordless
Once this is done, you can skip Step (1) and (2) in this section (see left nav bar) and move directly to setting up the SuperTokens core (Step 3).
Or, you can manually integrate SuperTokens by following the steps below.
Manual setup steps below
#
1) Install- ReactJS
- Angular
- Vue
npm i -s supertokens-auth-react
important
SuperTokens does not provide Angular components for pre built UI, but, there are two ways of implementing SuperTokens in your Angular frontend:
- Load SuperTokens's pre-built React UI components in your Angular app. Continue following the guide for this flow.
- Build your own UI. Click here to learn more.
Start by installing the SuperTokens ReactJS SDK and React:
npm i -s supertokens-auth-react react react-dom
You will also need to update compilerOptions
in your tsconfig.json
file with the following:
"jsx": "react",
"allowSyntheticDefaultImports": true,
important
SuperTokens does not provide Vue components for pre built UI, but, there are two ways of implementing SuperTokens in your Vue frontend:
- Load SuperTokens's pre-built React UI components in your Vue app. Continue following the guide for this flow.
- Build your own UI. Click here to learn more.
Start by installing the SuperTokens ReactJS SDK and React:
npm i -s supertokens-auth-react react react-dom
init
function#
2) Call the - ReactJS
- Angular
- Vue
import SuperTokens from 'supertokens-web-js';
import Session from 'supertokens-web-js/recipe/session';
SuperTokens.init({
appInfo: {
apiDomain: "<YOUR_API_DOMAIN>",
apiBasePath: "/auth",
appName: "...",
},
recipeList: [
Session.init(),
],
});
supertokens.init({
appInfo: {
apiDomain: "<YOUR_API_DOMAIN>",
apiBasePath: "/auth",
appName: "...",
},
recipeList: [
supertokensSession.init(),
],
});
import SuperTokens from 'supertokens-react-native';
SuperTokens.init({
apiDomain: "<YOUR_API_DOMAIN>",
apiBasePath: "/auth"
});
#
3) Setup Routing to show the login UI- ReactJS
- Angular
- Vue
Update your angular router so that all auth related requests load the auth
component
import { NgModule } from "@angular/core";
import { RouterModule, Routes } from "@angular/router";
const routes: Routes = [
{
path: "auth",
loadChildren: () => import("./auth/auth.module").then((m) => m.AuthModule),
},
{ path: "**", loadChildren: () => import("./home/home.module").then((m) => m.HomeModule) },
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule],
})
export class AppRoutingModule {}
Update your Vue router so that all auth related requests load the AuthView
component
import { createRouter, createWebHistory } from "vue-router";
import HomeView from "../views/HomeView.vue";
const router = createRouter({
history: createWebHistory(import.meta.env.BASE_URL),
routes: [
{
path: "/",
name: "home",
component: HomeView,
},
{
path: "/auth*",
name: "auth",
component: () => import("../views/AuthView.vue"),
},
],
});
export default router;
#
4) View the login UIYou can view the login UI by visiting /auth
.
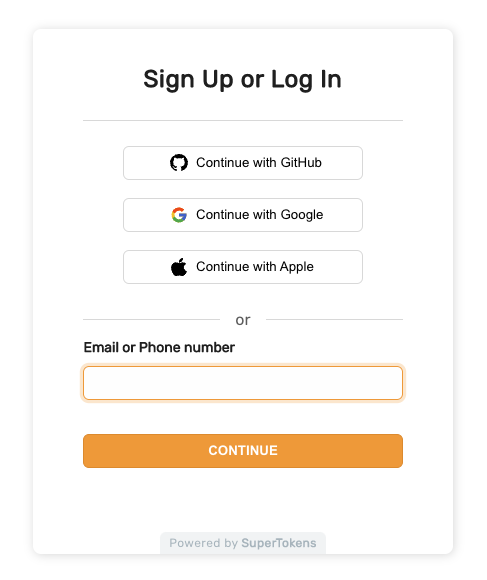
At this stage, you've successfully integrated your website with SuperTokens. The next section will guide you through setting up your backend.